SurveyMonkey is an online survey software. Analyzing open-ended survey responses uncovers trends in customer feedback, guiding business decisions and market research.
Welcome to the SurveyMonkey documentation for Dashbot! Integrating Dashbot into your SurveyMonkey application is quick and easy.
If you have any questions, comments, or suggestions, please feel free to contact us.
Set Up SurveyMonkey App
Create an App in SurveyMonkey:
- Log in to SurveyMonkey and go to the Developers Hub so we can add a new app and access the necessary credentials.
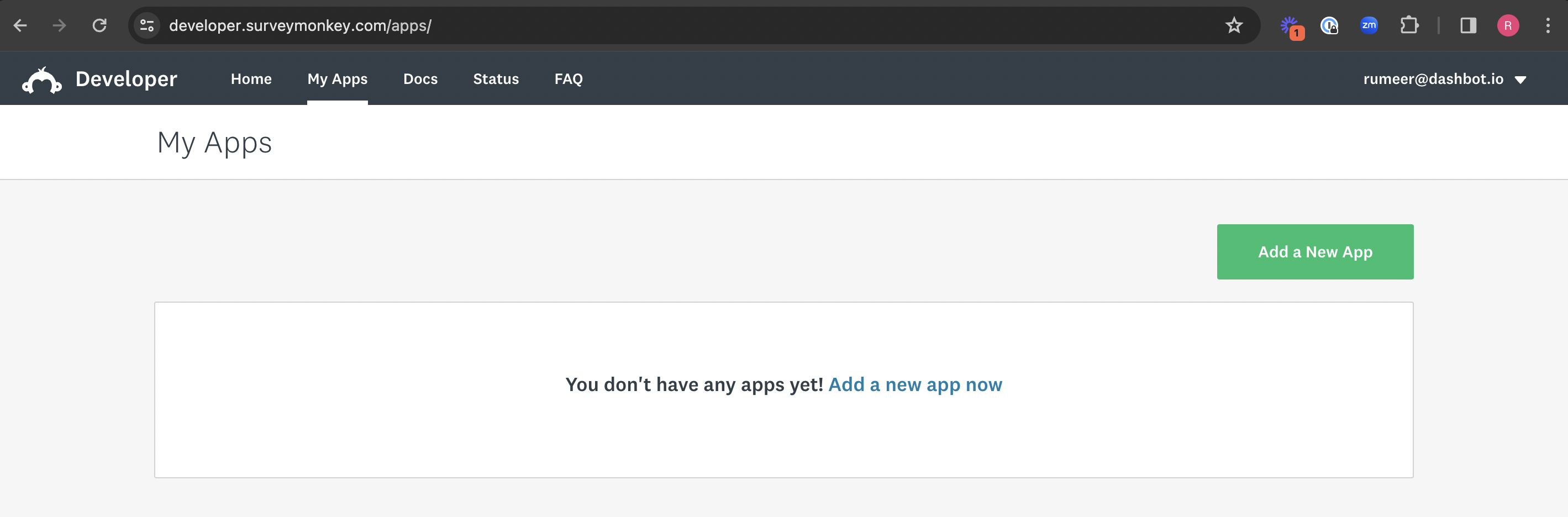
- Be sure to update the permissions of the app to all of the view settings so we can pass the survey response data along to Dashbot.
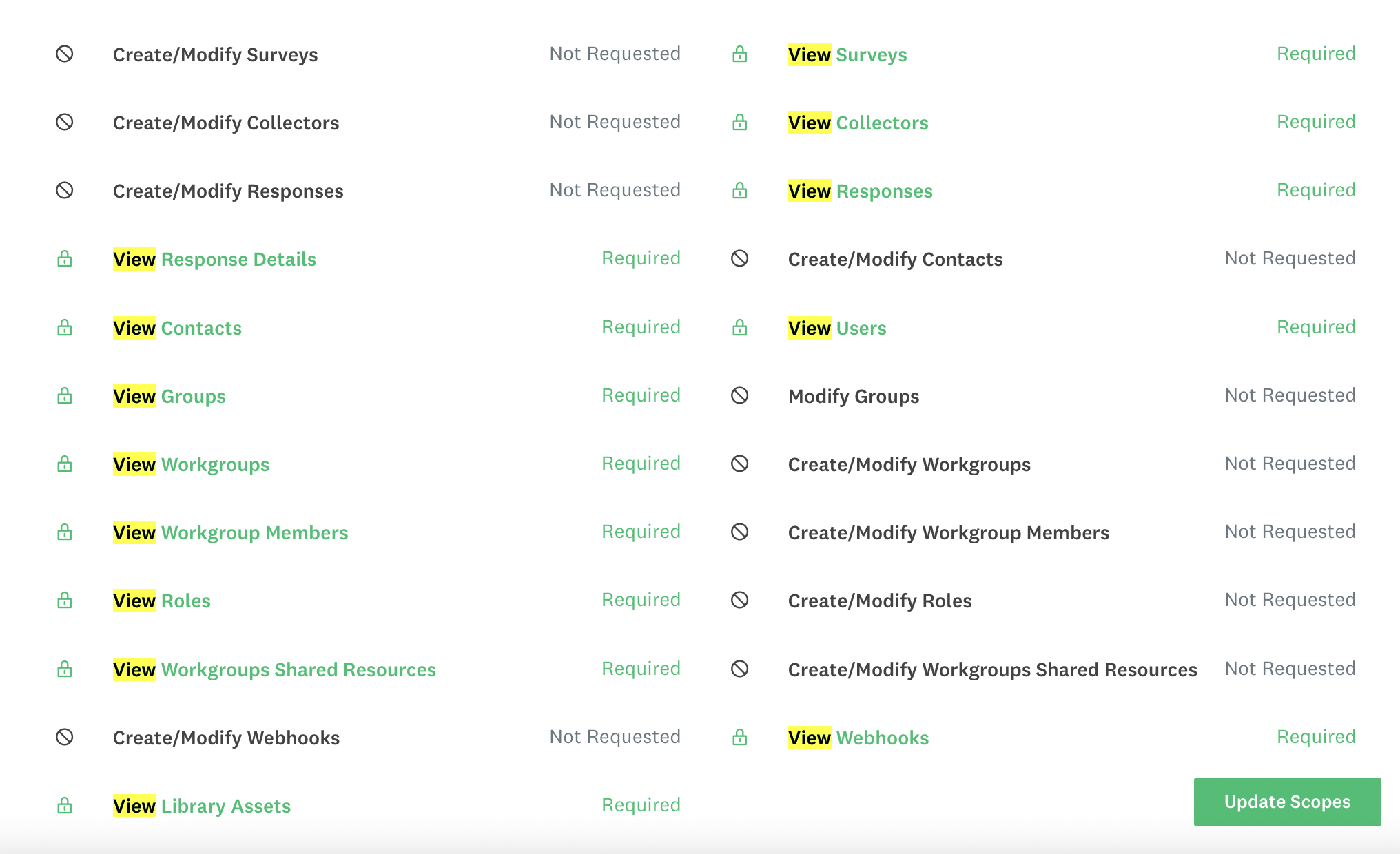
- Set your OAuth redirect URI in the app settings.
Example OAuth Link from survey Monkey:
https://api.surveymonkey.com/oauth/authorize?response_type=code&redirect_uri=https%3A%2F%2Fapi.surveymonkey.com%2Fapi_console%2Foauth2callback&client_id=SurveyMonkeyApiConsole%2Fstate=uniquestring
var SM_API_BASE = "https://api.surveymonkey.com";
var AUTH_CODE_ENDPOINT = "/oauth/authorize";
function oauth_dialog(client_id, redirect_uri) {
var auth_dialog_uri = SM_API_BASE + AUTH_CODE_ENDPOINT + "?redirect_uri=" + redirect_uri + "&client_id=" + client_id + "&response_type=" + code;
// Insert code here that redirects user to OAuth Dialog url
}
Replace YOUR_CLIENT_ID
and YOUR_REDIRECT_URI
with your actual values.
Exchange Authorization Code for Token:
After user authorization, capture the code from the redirect URI.
curl -i -X POST https://api.surveymonkey.com/oauth/token -d \
"client_secret=YOUR_CLIENT_SECRET \
&code=AUTH_CODE \
&redirect_uri=YOUR_REDIRECT_URI \
&client_id=YOUR_CLIENT_ID \
&grant_type=authorization_code"
var SM_API_BASE = "https://api.surveymonkey.com";
var ACCESS_TOKEN_ENDPOINT = "/oauth/token";
async function exchange_code_for_token(auth_code, client_secret, client_id, redirect_uri) {
var data = {
"client_secret": client_secret,
"code": auth_code,
"redirect_uri": redirect_uri,
"client_id": client_id,
"grant_type": "authorization_code"
}
access_token_uri = SM_API_BASE + ACCESS_TOKEN_ENDPOINT;
var access_token_response = await fetch(access_token_uri, {
"method": "POST",
"headers": {
"Content-Type": "application/json",
"Accept": "application/json",
"Authorization": "Bearer {access-token}"
},
"body": JSON.stringify(data)
});
var access_json = access_token_response.json();
return access_json["access_token"];
}
GET Survey Responses from SurveyMonkey
API Request for Survey Responses:
We are going to be leveraging the Survey Responses end point from SurveyMonkey. Specifically, we are looking at the getting all of the responses and questions from the survey returned to us.
https://api.surveymonkey.com/v3/collectors/{id}/responses/{id}/details
curl --request GET \
--url https://api.surveymonkey.com/v3/surveys/{id}/responses/{id}/details \
--header 'Accept: application/json' \
--header 'Authorization: Bearer {access-token}'
fetch("https://api.surveymonkey.com/v3/collectors/{id}/responses/{id}", {
"method": "GET",
"headers": {
"Accept": "application/json",
"Authorization": "Bearer {access-token}"
}
})
.then(response => {
console.log(response);
})
.catch(err => {
console.error(err);
});
POST responses to Dashbot
When your survey receives a response, post the data to the following endpoint:
https://tracker.dashbot.io/track?platform=universal&v=10.1.1-rest&type=incoming&apiKey=API_KEY_HERE
Make sure to set the ‘Content-Type’ header to ‘application/json’ and to replace API_KEY_HERE
with your api key.
You must send the data with the following fields:
- text – string – (required)
- userId – string – (required) – should be the SAME userId for both incoming and outgoing messages this is NOT the bot’s user ID
To review all optional fields see our API reference.
The data to POST should pass the following data:
{
"text": "Hi, bot",
"userId": "+14155551234",
"platformJson": {
"whateverJson": "any JSON specific to your platform can be stored here"
}
}
Sample cURL
curl -X POST -H "Content-Type: application/json"
-d '{"text":"Hi, bot","userId":"+14155551234","platformJson":{"whateverJson":"any JSON specific to your platform can be stored here"}}'
'https://tracker.dashbot.io/track?platform=sms&v=11.1.0-rest&type=incoming&apiKey=API_KEY'
Notice, you must replace the placeholder API_KEY_HERE
above with your api key.